😕 Why drop Apollo Client and urql for GraphQL Queries? #
In this post, we’ll look at how you can perform SvelteKit GraphQL queries using fetch only. That's right, there's no need to add Apollo client or urql to your Svelte apps if you have basic GraphQL requirements. We will get our GraphQL data from the remote API using just fetch functionality. You probably already know that the fetch API is available in client code . In SvelteKit, it is also available in load functions and server API routes. This means you can use the code we produce here to make GraphQL queries directly from page components or any server route.
We will use a currency API to pull the latest exchange rates for a few currencies, querying initially from a server API route. This will be super useful for a backend dashboard on your platform. You can use it to track payments received in foreign currencies, converting them back to your local currency, be that dollars, rupees, euros, pounds, or even none of those! This will be so handy if you are selling courses, merch, or even web development services globally . Once the basics are up and running, we will add another query from a client page and see how easy Svelte stores make it to update your user interface with fresh data.
If that all sounds exciting to you, then let’s not waste any time!
Please enable JavaScript to watch the video 📼
SvelteKit GraphQL Queries: Setup #
We'll start by creating a new project and installing packages:
pnpm dlx sv create sveltekit-graphql-fetch && cd $_pnpm installpnpm add -D @fontsource/source-sans-pro
When prompted, choose a Skeleton Project and answer Yes to TypeScript, ESLint and Prettier. We also added a self-hosted font package, which we will use later.
Please enable JavaScript to watch the video 📼
API Key #
We will be using the SWOP GraphQL API to pull the latest available currency exchange rates. To use the service, we will need an API key. There is a free developer tier, and they only ask for an email address when signing up. Let's go to the sign-up page now, sign up , confirm our email address and then make a note of our new API key.
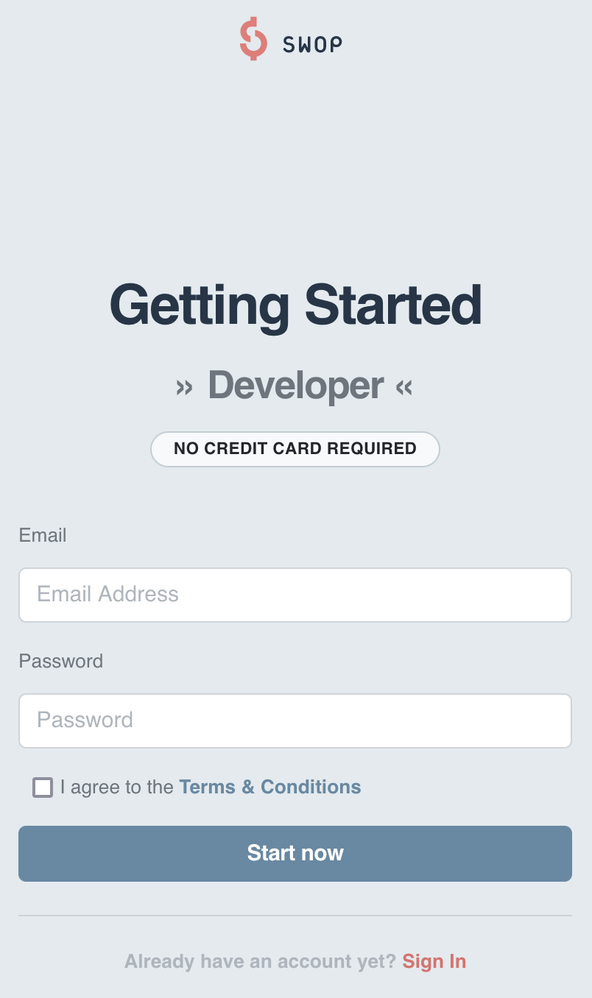
Configuring Environment Variables #
Create a .env
file in the project root folder containing
your API key:
SWOP_API_KEY="0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef"
With the preliminaries out of the way, let’s write our query.
🗳 Poll #
🧱 API Route #
To create a GraphQL query using fetch, basically all you need to do is create
a query object and a variables object, convert them to a string and then send
them as the body to the right API endpoint. We will use fetch
to do the sending as it is already included in SvelteKit though you could choose
axios
or some other package if you wanted to. In
addition to the body, we need to make sure we include the right auth headers (as
you would with Apollo client or urql).
That’s enough theory. If you want to do some more reading, Jason Lengstorf from Netlify wrote a fantastic article with plenty of extra details.
Let’s write some code. Create a file at src/routes/+page.server.ts
and paste in the following code:
1 import { SWOP_API_KEY } from '$env/static/private';2 import type { Query, QueryLatestArgs } from '$lib/generated/graphql';3 import { error } from '@sveltejs/kit';4 import type { Actions, PageServerLoad } from './$types';56 const query = `7 query latestQuery(8 $baseCurrency: String = "EUR"9 $quoteCurrencies: [String!]10 ) {11 latest(12 baseCurrency: $baseCurrency13 quoteCurrencies: $quoteCurrencies14 ) {15 baseCurrency16 quoteCurrency17 date18 quote19 }20 }21 `;2223 export const load: PageServerLoad = async () => {24 try {25 const variables: QueryLatestArgs = {26 baseCurrency: 'EUR',27 quoteCurrencies: ['CAD', 'GBP', 'IDR', 'INR', 'USD']28 };2930 const response = await fetch('https://swop.cx/graphql', {31 method: 'POST',32 headers: {33 'Content-Type': 'application/json',34 Authorization: `ApiKey ${SWOP_API_KEY}`35 },36 body: JSON.stringify({37 query,38 variables39 })40 });4142 const { data: responseData }: { data: Query } = await response.json();4344 return { ...responseData };45 } catch (error) {46 console.error(`Error in load function for /: ${error}`);47 }48 };
What this Code Does #
You can see in lines 6
– 21
we define the GraphQL
query. This uses regular GraphQL syntax. Just below, we define our query variables.
If you are making a different query which does not need any variables, be sure
to include an empty variables object.
In line 30
you see we make a fetch request to the
SWOP API. Importantly, we include the Content-Type
header, set to application/json
in line 33
. The rest of the file just processes the response and relays it to the
client.
Let’s create a store to save retrieved data next.
🛍 Store #
We will create a store as our “single source of truth”. Stores are an idiomatic, Svelte way of sharing app state between components. We won't go into much detail here, and you can learn more about Svelte stores in the Svelte tutorial .
To build the store, all we need to do is create the following file. Let's do
that now, pasting the content below into src/lib/shared/stores/rates.ts
(you will need to create new folders):
1 import { writable } from 'svelte/store';23 const rates = writable([]);45 export { rates as default };
Next, we can go client side to make use of SvelteKit GraphQL queries using fetch only.
🖥 Initial Client Code: SvelteKit GraphQL queries using fetch #
We are using TypeScript in this project, but very little, so hopefully you can
follow along even though you are not completely familiar with TypeScript.
Replace the content of src/routes/+page.svelte
with the following:
1 <script lang="ts">2 import rates from '$lib/shared/stores/rates';3 import '@fontsource/source-sans-pro/latin.css';4 import type { ActionData, PageData } from './$types';56 let { data }: { data: PageData } = $props();78 $effect(() => {9 if (data.latest) {10 rates.set(data.latest);11 }12 });13 </script>1415 <main class="container">16 <div class="heading">17 <h1>FX Rates</h1>18 </div>19 <ul class="content">20 {#each $rates as { baseCurrency, quoteCurrency, date, quote }}21 <li>22 <h2>{`${baseCurrency}\${quoteCurrency}`}</h2>23 <dl>24 <dt>25 {`1 ${baseCurrency}`}26 </dt>27 <dd>28 <span class="rate">29 {quote.toFixed(2)}30 {quoteCurrency}31 </span>32 <details><summary>More information...</summary>Date: {date}</details>33 </dd>34 </dl>35 </li>36 {/each}37 </ul>3839 <form method="POST">40 <span class="screen-reader-text"41 ><label for="additional-currency">Additional Currency</label></span42 >43 <input44 required45 id="additional-currency"46 name="currency"47 placeholder="AUD"48 title="Add another currency"49 type="text"50 />51 <button type="submit">Add currency</button>52 </form>53 </main>
What are we Doing Here? #
In line 6
, we import the data
parameter from the page load function (we will create this in a moment). This
contains the initial rate data we got from the GraphQL query.
At line 2
, we import the store we just
created. Next, we actually make use of the store. We add the query result to
the store in line 10
. We will actually render
whatever is in the store, rather than the result of the query directly. The
benefit of doing it that way is that we can easily update what is rendered by
adding another other currency pair to the store (without any complex logic for
merging what is already rendered with new query results). You will see this
shortly.
Optional Styling #
This should all work as is. Optionally add a little style before continuing:
src/routes/+page.svelte
— click to expand code.
51 <style>52 :global body {53 margin: 0px;54 }5556 .container {57 display: flex;58 flex-direction: column;59 background: #ff6b6b;60 min-height: 100vh;61 color: #1a535c;62 font-family: 'Source Sans Pro';63 }6465 .content {66 margin: 3rem auto 1rem;67 width: 50%;68 border-radius: 1rem;69 border: #f7fff7 solid 1px;70 }7172 .heading {73 background: #f7fff7;74 text-align: center;75 width: 50%;76 border-radius: 1rem;77 border: #1a535c solid 1px;78 margin: 3rem auto 0rem;79 padding: 0 1.5rem;80 }8182 h1 {83 color: #1a535c;84 }8586 ul {87 background: #1a535c;88 list-style-type: none;89 padding: 1.5rem;90 }9192 li {93 margin-bottom: 1.5rem;94 }9596 h2 {97 color: #ffe66d;98 margin-bottom: 0.5rem;99 }100101 dl {102 background-color: #ffe66d;103 display: flex;104 margin: 0.5rem 3rem 1rem;105 padding: 1rem;106 border-radius: 0.5rem;107 border: #ff6b6b solid 1px;108 }109110 .rate {111 font-size: 1.25rem;112 }113 dt {114 flex-basis: 15%;115 padding: 2px 0.25rem;116 }117118 dd {119 flex-basis: 80%;120 flex-grow: 1;121 padding: 2px 0.25rem;122 }123124 form {125 margin: 1.5rem auto 3rem;126 background: #4ecdc4;127 border: #1a535c solid 1px;128 padding: 1.5rem;129 border-radius: 1rem;130 width: 50%;131 }132 input {133 font-size: 1.563rem;134 border-radius: 0.5rem;135 border: #1a535c solid 1px;136 background: #f7fff7;137 padding: 0.25rem 0.25rem;138 margin-right: 0.5rem;139 width: 6rem;140 }141 button {142 font-size: 1.563rem;143 background: #ffe66d;144 border: #1a535c solid 2px;145 padding: 0.25rem 0.5rem;146 border-radius: 0.5rem;147 cursor: pointer;148 }149150 .screen-reader-text {151 border: 0;152 clip: rect(1px, 1px, 1px, 1px);153 clip-path: inset(50%);154 height: 1px;155 margin: -1px;156 width: 1px;157 overflow: hidden;158 position: absolute !important;159 word-wrap: normal !important;160 }161162 @media (max-width: 768px) {163 .content,164 form,165 .heading {166 width: auto;167 margin: 1.5rem;168 }169 }170 </style>
OK, let’s take a peek at what we have so far by going to localhost:5173/
.
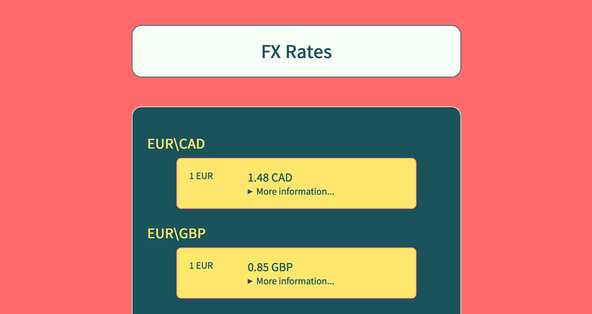
🚀 SvelteKit GraphQL queries using fetch: Updating the Store #
Finally, we will look at how updating the store updates the user interface. We
already have a form in src/routes/+page.svelte
(lines 35
– 48
), but it is not
yet wired up. We already have a name
attribute
set on the input. We can use this, now, in our action code, to pull in whatever
the user enters. Update src/routes/+page.server.ts
:
1 import { SWOP_API_KEY } from '$env/static/private';2 import type { Query, QueryLatestArgs } from '$lib/generated/graphql';3 import { error } from '@sveltejs/kit';4 import type { Actions, PageServerLoad } from './$types';56 const query = `7 query latestQuery(8 $baseCurrency: String = "EUR"9 $quoteCurrencies: [String!]10 ) {11 latest(12 baseCurrency: $baseCurrency13 quoteCurrencies: $quoteCurrencies14 ) {15 baseCurrency16 quoteCurrency17 date18 quote19 }20 }21 `;2223 export const actions: Actions = {24 default: async ({ request }) => {25 try {26 const form = await request.formData();27 const currency = form.get('currency');2829 if (typeof currency === 'string') {30 const variables: QueryLatestArgs = {31 baseCurrency: 'EUR',32 quoteCurrencies: [currency]33 };3435 const response = await fetch('https://swop.cx/graphql', {36 method: 'POST',37 headers: {38 'Content-Type': 'application/json',39 Authorization: `ApiKey ${SWOP_API_KEY}`40 },41 body: JSON.stringify({42 query,43 variables44 })45 });4647 const responseData: { data: Query } = await response.json();48 const rate = responseData.data.latest[0];4950 return { rate };51 }52 return undefined;53 } catch (err: unknown) {54 const message = `Error in /login form: ${err as string}`;55 console.error(message);56 return error(500, message);57 }58 }59 };6061 export const load: PageServerLoad = async () => {62 try {63 const variables: QueryLatestArgs = {64 baseCurrency: 'EUR',65 quoteCurrencies: ['CAD', 'GBP', 'IDR', 'INR', 'USD']66 };6768 const response = await fetch('https://swop.cx/graphql', {69 method: 'POST',70 headers: {71 'Content-Type': 'application/json',72 Authorization: `ApiKey ${SWOP_API_KEY}`73 },74 body: JSON.stringify({75 query,76 variables77 })78 });7980 const { data: responseData }: { data: Query } = await response.json();8182 return { ...responseData };83 } catch (error) {84 console.error(`Error in load function for /: ${error}`);85 }86 };
In lines 26
– 27
, we read in the
form data, identifying the field we need using the value of its name field (currency
). After that, the code is not that different from the load function that
already existed in this file. The main difference is that we query for and
return a single rate, rather than an array of rates, as in the load function.
We need to read this in the client, Svelte, file. Update src/routes/+page.svelte
with these lines so, once the user submits the form, we trigger a store update:
1 <script lang="ts">2 import rates from '$lib/shared/stores/rates';3 import '@fontsource/source-sans-pro/latin.css';4 import type { ActionData, PageData } from './$types';56 let { data, form }: { data: PageData; form: ActionData } = $props();78 $effect(() => {9 if (data.latest) {10 rates.set(data.latest);11 }12 });1314 $effect(() => {15 if (16 form?.rate &&17 !$rates.find(({ quoteCurrency }) => quoteCurrency === form.rate.quoteCurrency)18 ) {19 rates.set([...$rates, form.rate]);20 }21 });22 </script>2324 <main class="container">25 <div class="heading">26 <h1>FX Rates</h1>27 </div>28 <ul class="content">29 {#each $rates as { baseCurrency, quoteCurrency, date, quote }}30 <li>31 <h2>{`${baseCurrency}\${quoteCurrency}`}</h2>32 <dl>33 <dt>34 {`1 ${baseCurrency}`}35 </dt>36 <dd>37 <span class="rate">38 {quote.toFixed(2)}39 {quoteCurrency}40 </span>41 <details><summary>More information...</summary>Date: {date}</details>42 </dd>43 </dl>44 </li>45 {/each}46 </ul>4748 <form method="POST">49 <span class="screen-reader-text"50 ><label for="additional-currency">Additional Currency</label></span51 >52 <input53 required54 id="additional-currency"55 name="currency"56 placeholder="AUD"57 title="Add another currency"58 type="text"59 />60 <button type="submit">Add currency</button>61 </form>62 </main>
Try this out yourself, adding a couple of currencies. The interface should update straight away.
🙌🏽 SvelteKit GraphQL queries using fetch: What Do You Think? #
In this post, we learned:
- how to do SvelteKit GraphQL queries using fetch instead of Apollo client or urql,
- a way to get up-to-date currency exchange rate information into your site backend dashboard for analysis, accounting, and so many other uses,
- how stores can be used in Svelte to update state.
See the follow-up post in which we automatically generate TypeScript types for the GraphQL API. Type generation is not too onerous in this example, though you can see how to do it automatically for more intricate GraphQL APIs, getting free autocompletion and help to spot code errors.
There are some restrictions on the base currency in SWOP's developer mode. The maths (math) to convert from EUR to the desired base currency isn't too complicated, though. You could implement a utility function to do the conversion in the API route file. If you do find the service useful, or and expect to use it a lot, consider supporting the project by upgrading your account.
Extensions #
As an extension you might consider pulling historical data from the SWOP API, this is not too different, to the GraphQL query above. Have play in the SWOP GraphQL Playground to discover more of the endless possibilities . Finally, you might also find the Purchasing Power API handy if you are looking at currencies. This is not a GraphQL API, though it might be quite helpful for pricing your courses in global economies you are not familiar with.
Is there something from this post you can leverage for a side project or even client project? I hope so! Let me know if there is anything in the post that I can improve on, for anyone else creating this project. You can leave a comment below, @ me on Twitter or try one of the other contact methods listed below.
You can see the full code for this SvelteKit GraphQL queries using fetch project on the Rodney Lab Git Hub repo .
🏁 SvelteKit GraphQL queries using fetch: Summary #
Do you need to use Apollo Client or urql to run GraphQL queries in SvelteKit? #
- SvelteKit makes fetch available in load functions and on endpoints. This is a great alternative to Apollo Client and urql when you want to get up and running quickly on your new project. Although you won't have access to the caching features provided by Apollo Client and urql if you use just fetch, SvelteKit does make it easy to create a cache using stores. The advantages of using fetch are you keep your app lighter, with fewer dependencies, and also that the API is very stable. This saves you refactoring code as APIs change.
How do you use fetch for GraphQL queries in SvelteKit? #
- Using fetch for GraphQL queries is as simple as creating a query and variables object and sending those to your endpoint. In the case your query does not need any variables, you will still need to include an albeit empty variable object in the request. The query variable is the GraphQL query (or mutation) using regular GraphQL syntax, as a string. We use the POST method to send the query to our external API. Importantly, we include the 'Content-Type': 'application/json' HTTP header in the request.
Where can I find some example code using fetch for GraphQL queries in SvelteKit? #
- In this tutorial, we run through an example using an FX rate GraphQL API to fetch data. We make GraphQL calls both from the client code and our API endpoints. There is also a link to the complete tutorial project code on GitHub at the bottom of the article. You can follow along, building the project out yourself, or just jump straight to the GitHub repo.
🙏🏽 SvelteKit GraphQL queries using fetch: Feedback #
Have you found the post useful? Do you have your own methods for solving this problem? Let me know your solution. Would you like to see posts on another topic instead? Get in touch with ideas for new posts. Also, if you like my writing style, get in touch if I can write some posts for your company site on a consultancy basis. Read on to find ways to get in touch, further below. If you want to support posts similar to this one and can spare a few dollars, euros or pounds, please consider supporting me through Buy me a Coffee.
🔥 Here's something to help you keep your SvelteKit GraphQL apps lean.
— Rodney (@askRodney) September 27, 2021
See how you can query
#GraphQL endpoints using just fetch... no urql, no Apollo client!
Also handle state with ❤️ Svelte stores.
Hope you find this useful!https://t.co/D1rLmh229H #sveltekit @SvelteSociety pic.twitter.com/087gRALZxq
Finally, feel free to share the post on your social media accounts for all your followers who will find it useful. As well as leaving a comment below, you can get in touch via @askRodney on Twitter and also askRodney on Telegram . Also, see further ways to get in touch with Rodney Lab. I post regularly on SvelteKit as well as other topics. Also, subscribe to the newsletter to keep up-to-date with our latest projects.